Streamlining Application Detection in Intune and MECM with PowerShell
In the dynamic world of IT management, tools like Microsoft Intune and Microsoft Endpoint Configuration Manager (MECM) are vital for efficiently managing applications across a multitude of Windows devices. However, managing and detecting specific applications in diverse environments can often be challenging. This is where template PowerShell scripts like PSIntuneWinDetection
become invaluable, offering refined and precise application detection and management capabilities.
Unveiling the PSIntuneWinDetection Script
The PSIntuneWinDetection
PowerShell function is crafted to simplify detecting specific applications installed on Windows systems. This script is an asset in environments where you're dealing with varied installations, be it 32-bit, 64-bit, or user-specific software.
Core Features
- Accurate Application Detection: Searches for applications based on display name and version.
- Architecture Compatibility: Equipped to check both 64-bit and 32-bit registry paths.
- User Context Awareness: Capable of detecting installations in user-specific contexts (
HKCU
registry hive).
The Script
The script functions by creating an UninstallInfo
class that stores vital information about installed applications. It uses the Get-UninstallInfo
function to gather installation data from specific registry paths, addressing both general and user-specific installations.
# App and version we are looking for
$AppDisplayName = "your_app_name" # Example: "Microsoft Visual Studio Code"
$AppDisplayVersion = "your_app_version" # Example: "1.84.2"
function PSIntuneWinDetection {
param($SearchFor, [switch]$Wow6432Node, [switch]$userContext)
class UninstallInfo {
[string]$GUID
[string]$Publisher
[string]$DisplayName
[string]$DisplayVersion
[string]$InstallLocation
[string]$InstallDate
[string]$UninstallString
[string]$Wow6432Node
[string]$UserContext
}
function Get-UninstallInfo {
param($Path, [string]$Node = 'No', [string]$UserCtx = 'No')
Get-ChildItem $Path | ForEach-Object {
$info = [UninstallInfo]::new()
$info.GUID = $_.pschildname
$info.Publisher = $_.GetValue('Publisher')
$info.DisplayName = $_.GetValue('DisplayName')
$info.DisplayVersion = $_.GetValue('DisplayVersion')
$info.InstallLocation = $_.GetValue('InstallLocation')
$info.InstallDate = $_.GetValue('InstallDate')
$info.UninstallString = $_.GetValue('UninstallString')
$info.Wow6432Node = $Node
$info.UserContext = $UserCtx
$info
}
}
$results = [System.Collections.Generic.List[UninstallInfo]]::new()
# Default registry path
[UninstallInfo[]]$defaultInfo = Get-UninstallInfo 'HKLM:\SOFTWARE\Microsoft\Windows\CurrentVersion\Uninstall'
$results.AddRange($defaultInfo)
# Additional paths based on switches
if ($Wow6432Node) {
[UninstallInfo[]]$wow6432Info = Get-UninstallInfo 'HKLM:\SOFTWARE\Wow6432Node\Microsoft\Windows\CurrentVersion\Uninstall' 'Yes'
$results.AddRange($wow6432Info)
}
if ($userContext) {
[UninstallInfo[]]$userInfo = Get-UninstallInfo 'HKCU:\SOFTWARE\Microsoft\Windows\CurrentVersion\Uninstall' 'No' 'Yes'
$results.AddRange($userInfo)
}
# Sorting and filtering results
$results | Sort-Object DisplayName | Where-Object { $_.DisplayName -match $SearchFor }
}
# Usage example:
$appDetected = PSIntuneWinDetection -SearchFor $AppDisplayName -Wow6432Node -userContext | Where-Object { $_.DisplayVersion -eq $AppDisplayVersion }
if ($appDetected) {
return "installed"
}
The culmination of the script is an aggregation of this data, sorted and filtered based on the application's display name and version.
Integration with Intune and MECM
Enhancing Intune's Functionality
Microsoft Intune, a part of Microsoft's Endpoint Manager, is a predominantly cloud-based tool used for managing applications on remote devices. While Intune offers robust deployment tools, PSIntuneWinDetection
adds an essential layer of precision in verifying the installation of specific Windows-based application versions, vital for compliance and update management.
Augmenting MECM's Capabilities
MECM, a comprehensive on-premises solution for managing Windows-based systems, excels in inventory management and software distribution. The integration of PSIntuneWinDetection
within MECM can significantly improve its application detection processes. This script offers a swift, efficient method to confirm the presence and versions of applications, ensuring deployment accuracy.
Example PSIntuneWinDetection Output:
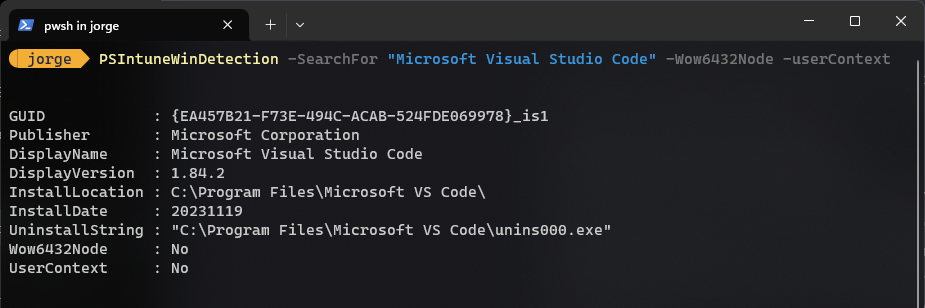
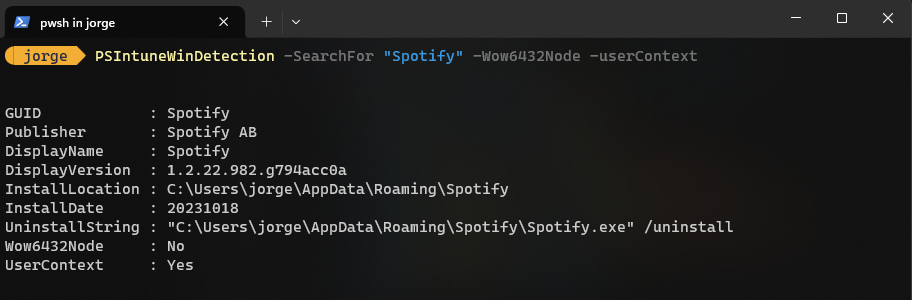
Explore More
Interested in exploring PSIntuneWinDetection
further or contributing changes? Visit the GitHub repository for additional insights and code:
PSIntuneWinDetection on GitHub.
Wrapping Up
PSIntuneWinDetection
is a testament to the power of PowerShell in augmenting application management within Intune and MECM. By providing a detailed approach to application detection, it ensures a more precise deployment and update process in both cloud and on-premise environments. As IT landscapes grow increasingly complex, such tools are indispensable in maintaining oversight and control.
Hope this helps.
> jorgeasaurus